pythonを使ってテキストマイニングを行なっていると、もっと多くのデータを取得して、テキストマイニングを行いたい、と思うようになるのではないでしょうか
APIの認証を受けていれば、大量のtwitterデータを使ってテキストマイニングを行うことができます
この記事では、pythonでtwitterデータからテキストマイニングを行う方法について解説していきたいと思います
pythonでtwitterからツイートを取得したい
twitterデータからできストマイニングを行いたい
分析用のデータが欲しい
といった方に向けて、書いていきます!
以下の記事では、pythonでtwitterを使う方法をまとめて解説しているので、参考にしてみてください
短時間でpythonを学びたい方は、こちらがおすすめ
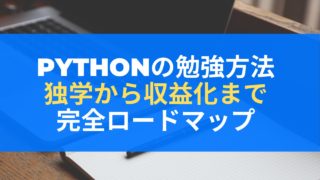
Contents
pythonでtwitterデータからテキストマイニングを行う
twitter APIを使うことで、twitterデータを収集することができます
収集したデータをもとにテキストマイニングをおこなうと以下のようになります
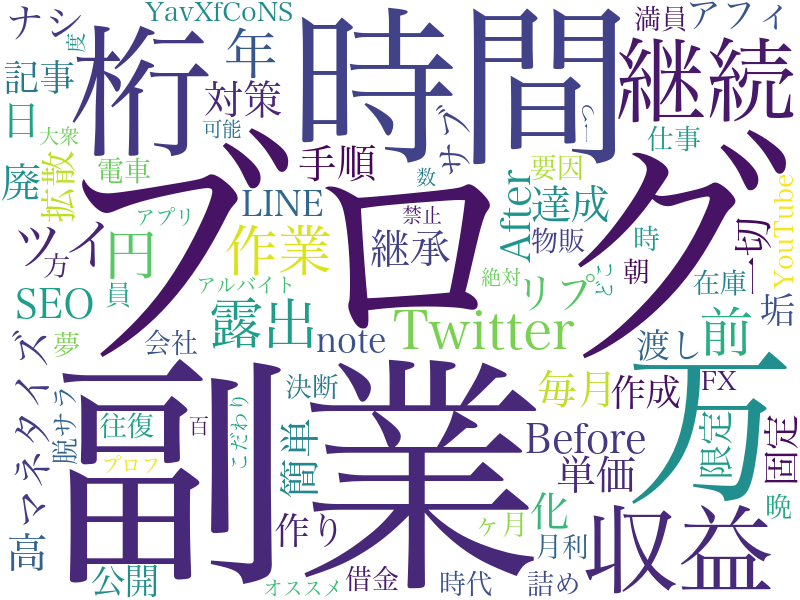
APIの認証が通っていると、pythonを使ってのtwitter操作の幅が広がるので、twitter APIの認証を通しておくのがおすすめです
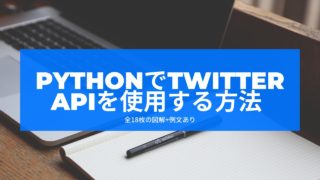
実際のコード
import tweepy
from datetime import datetime,timezone
import pytz
import pandas as pd
import collections
import matplotlib.pyplot as plt
from wordcloud import WordCloud
CONSUMER_KEY = ''
CONSUMER_SECRET = ''
ACCESS_TOKEN = ''
ACCESS_SECRET = ''
auth = tweepy.OAuthHandler(CONSUMER_KEY, CONSUMER_SECRET)
auth.set_access_token(ACCESS_TOKEN, ACCESS_SECRET)
api = tweepy.API(auth)
search_results = api.search_tweets(q="", result_type="recent",tweet_mode='extended',count=5)
tw_data = []
for tweet in search_results:
#tweet_dataの配列に取得したい情報を入れていく
tw_data.append([
tweet.id,
tweet_time,
tweet.full_text,
tweet.favorite_count,
tweet.retweet_count,
tweet.user.id,
tweet.user.screen_name,
tweet.user.name,
tweet.user.description,
tweet.user.friends_count,
tweet.user.followers_count,
create_account_time,
tweet.user.following,
tweet.user.profile_image_url,
tweet.user.profile_background_image_url,
tweet.user.url
])
#取り出したデータをpandasのDataFrameに変換
#CSVファイルに出力するときの列の名前を定義
labels=[
'ツイートID',
'ツイート時刻',
'ツイート本文',
'いいね数',
'リツイート数',
'ID',
'ユーザー名',
'アカウント名',
'自己紹介文',
'フォロー数',
'フォロワー数',
'アカウント作成日時',
'自分のフォロー状況',
'アイコン画像URL',
'ヘッダー画像URL',
'WEBサイト'
]
#tw_dataのリストをpandasのDataFrameに変換
df = pd.DataFrame(tw_data,columns=labels)
df1=df.iat[2,2]
df2=df.iat[3,2]
tw_text=df1 + df2
f=open('text.txt','w')
f.write(tw_text)
f.close
f= open("/text.txt", 'r', encoding='UTF-8')
text=f.read()
f.close()
word_list=[]
while node:
word_type = node.feature.split(',')[0]
if word_type in ["名詞",'代名詞']:
word_list.append(node.surface)
node=node.next
word_chain=' '.join(word_list)
c=collections.Counter(word_list)
font_path='/System/Library/Fonts/ヒラギノ明朝 ProN.ttc'
words = ['https','t','co','自民','し','w','そう', 'ない', 'いる', 'する', 'まま', 'よう', 'てる', 'なる', 'こと', 'もう', 'いい', 'ある', 'ゆく', 'れる', 'ん', 'の']
result = WordCloud(width=800, height=600, background_color='white',
font_path=font_path,regexp=r"[\w']+",
stopwords=words).generate(word_chain)
result.to_file("./wordcloud_sample1.png")
print(c.most_common(20))
fig = plt.subplots(figsize=(8, 10))
コード解説
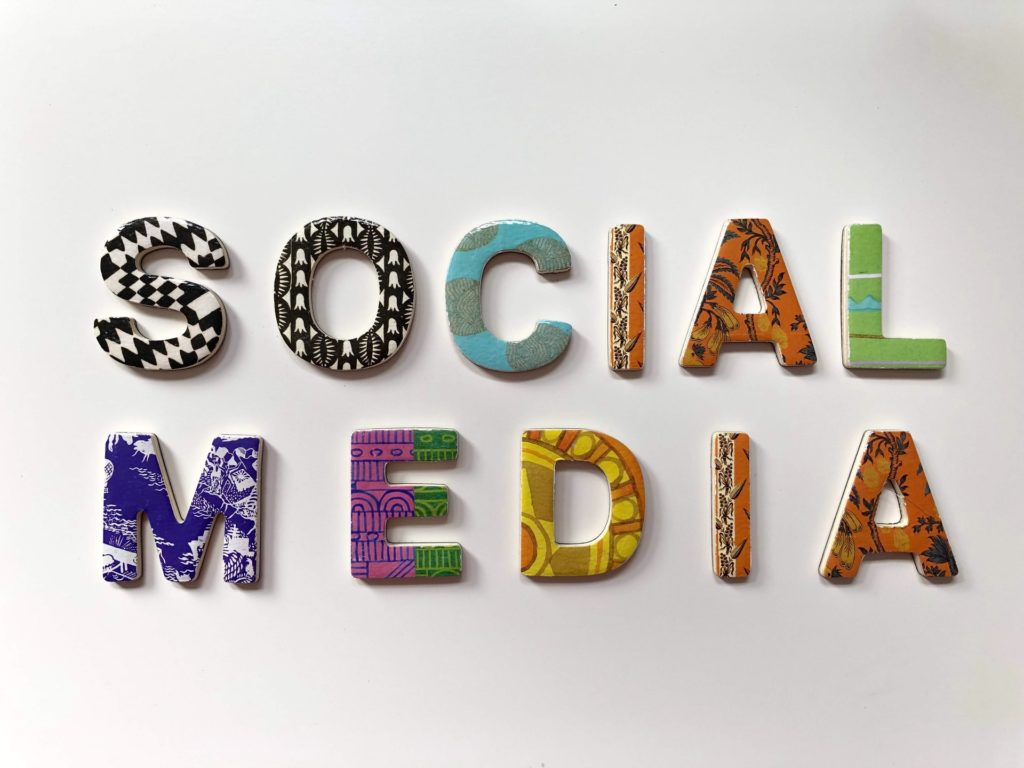
twitter API認証からテキストマイニングまでのコード解説をしていきます
コードを少し変更することで、さらに応用をすることができます
twitter APIの認証
import tweepy
CONSUMER_KEY = ''
CONSUMER_SECRET = ''
ACCESS_TOKEN = ''
ACCESS_SECRET = ''
auth = tweepy.OAuthHandler(CONSUMER_KEY, CONSUMER_SECRET)
auth.set_access_token(ACCESS_TOKEN, ACCESS_SECRET)
api = tweepy.API(auth)
pythonでtwitterを扱う場合には、必ずといって良いほど、上記のコードを入力していきます
これはtwitter APIの認証です
まずはこれを記載しておかないと、pythonでtwittreを操作することができません
検索キーワードの設定
#tweepyで検索を行う
search_results = api.search_tweets(q="", result_type="recent",tweet_mode='extended',count=5)
「api.search_tweets」でqにキーワードを入力することで検索をすることができます
#例
#tweepyで検索を行う
search_results = api.search_tweets(q="大谷翔平", result_type="recent",tweet_mode='extended',count=5)
result_typeは3種類です
- “recent”:時系列で最新ツイートを検索
- “popular”:人気のあるツイートを検索
- “mixed”:上記を混ぜたもの
となっています
デフォルトでは、”recent”になっているので、変更する場合には、「result_type=””」で指定する必要があります
tweet_mode=’extended’では、ツイート内容の全文を取得するための記載です
count=5は取得するツイート件数を決めています
データフレームの作成
tw_data = []#tw_dataという空のリストを作成
#ここからツイート情報を取得
for tweet in search_results:
tw_data.append([
tweet.id,
tweet_time,
tweet.full_text,
tweet.favorite_count,
tweet.retweet_count,
tweet.user.id,
tweet.user.screen_name,
tweet.user.name,
tweet.user.description,
tweet.user.friends_count,
tweet.user.followers_count,
create_account_time,
tweet.user.following,
tweet.user.profile_image_url,
tweet.user.profile_background_image_url,
tweet.user.url
])
#取り出したデータをpandasのDataFrameに変換
#CSVファイルに出力するときの列の名前を定義
labels=[
'ツイートID',
'ツイート時刻',
'ツイート本文',
'いいね数',
'リツイート数',
'ID',
'ユーザー名',
'アカウント名',
'自己紹介文',
'フォロー数',
'フォロワー数',
'アカウント作成日時',
'自分のフォロー状況',
'アイコン画像URL',
'ヘッダー画像URL',
'WEBサイト'
]
#tw_dataのリストをpandasのDataFrameに変換
df = pd.DataFrame(tw_data,columns=labels)
取得したツイートをデータフレームに変換します
その後テキストファイルに保存をしていきますが、その時に「ツイート本文」が含まれているセルだけをテキストファイルとしていきます
df1=df.iat[2,2]
df2=df.iat[3,2]
tw_text=df1 + df2
f=open('text.txt','w')
f.write(tw_text)
f.close
iatで指定をしていますが、ツイート本文全てを選択し、結合・テキストファイル保存をしておきます
テキストマイニングを行う
word_list=[]
while node:
word_type = node.feature.split(',')[0]
if word_type in ["名詞",'代名詞']:
word_list.append(node.surface)
node=node.next
word_chain=' '.join(word_list)
c=collections.Counter(word_list)
font_path='/System/Library/Fonts/ヒラギノ明朝 ProN.ttc'
words = ['https','t','co','自民','し','w','そう', 'ない', 'いる', 'する', 'まま', 'よう', 'てる', 'なる', 'こと', 'もう', 'いい', 'ある', 'ゆく', 'れる', 'ん', 'の']
result = WordCloud(width=800, height=600, background_color='white',
font_path=font_path,regexp=r"[\w']+",
stopwords=words).generate(word_chain)
result.to_file("./wordcloud_sample1.png")
print(c.most_common(20))
fig = plt.subplots(figsize=(8, 10))
wordcloudを実行、画像として出力という流れになっています
wordcloudで抽出するのは、「名詞と代名詞」にしていますが、〇〇詞を変えることで、その他のものも抽出することができます
また、wordsでは除外するワードを指定しています
twitterのツイートではURLが貼られていることもあるので、それを除外します
テキストマイニングとは
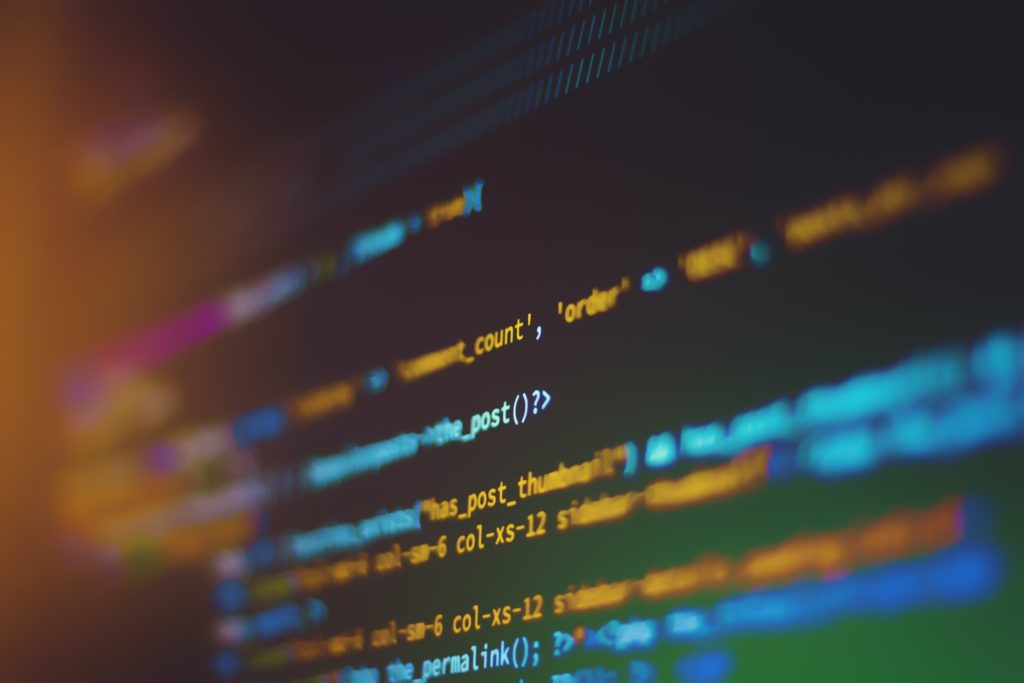
テキストマイニングとは、文字列を対象としたデータマイニングのことを指しています
通常の文章からなるデータを単語や文節で区切り、単語などの出現頻度・共出現の相関・時系列などを解析することができます
pythonでテキストマイニングを行うには
pythonでテキストマイニングを行うには、wordcloudと呼ばれるライブラリを使用します
wordcloudはテキストマイニングを行なった結果を可視化するためのものです
wordcloudをpythonで使用するには、インポートする必要があります
ターミナルもしくはコマンドプロンプトで以下のように入力をします
pip install python
wordcloudを使っていく
wordcloudを行うには、文字列が入力されているテキストファイルが必要になります
今回の場合は、ツイート本文が記載されている「text.txt」が該当します
wordcloudのパラメータを入力する
wordcloudのパラメータは左から順番に、
- 作成する画像の横幅
- 作成する画像の縦幅
- 背景色
- フォントファイルのパス
- 表示される文字の正規表現
- 除外する単語
- 文字列からwordcloudを作成
となります
result = WordCloud(width=800, height=600, background_color='white',
font_path=font_path,regexp=r"[\w']+",
stopwords=words).generate(word_chain)
まとめ
- pythonでtwitterからデータ収集するならAPI認証が必須
- pythonでテキストマイニングするならwordcloudがおすすめ